Exercise 101 Solution
We consider the three Pythons dictionaries which includes all the computer hardwares:
dicPC = {"HP": 11, "Acer": 7, "Lenovo": 17, "Del": 23}
dicPhone = {"Sumsung": 22, "Iphone": 9, "Other": 13}
dicTablet = {"Sumsung": 15, "Other": 13}
Write a Python program that combines by concatenating these three dictionaries into one.
Exrcise 102 Solution
We consider the following dictionary whose keys are the names of the students and the key values
are the general averages obtained by passing the final exam:
students = {"student_1" : 13 , "student_2" : 17 , "student_3" : 9 , "student_4" : 15 ,
"student_5" : 8 , "student_6" : 14 , "student_7" : 16 , "student_8" : 12 ,
"student_9" : 13 , "student_10" : 15 , "student_11" : 14 , "student_112" : 9 ,
"student_13" : 10 , "student_14" : 12 , "student_15" : 13 , "student_16" : 7 ,
"student_17" : 12 , "student_18" : 15 , "student_19" : 9 , "student_20" : 17 ,}
Write a Python program that partitions this dictionary into two sub-dictionaries:
- admittedStudents whose keys are the admitted students and the key values are the averages obtained (average
greater than or equal to 10).
- nonAdmittedStudents whose keys are non-admitted students and the key values are the averages obtained
(average less than or equal to 10).
Exercise 103 || Solution
Write a Python program that creates from a keyboard-typed integer n,
a dictionary whose keys are integers from 1 to n and key values are their squares.
Example for n = 7 the dictionary will be of the form:
{1: 1, 2: 4, 3: 9, 4:16, 5:25, 6:36, 7:49}
Exercise 104 || Solution
Write a program in Python that asks the user to enter a string,
and return him a dictionary whose keys are the characters in the string entered
and the values are the number of occurrences of the characters in the string.
Example if the entered string is s = 'language', the program returns the dictionary:
d = {'l': 1, 'a': 2, 'n': 1, 'g': 2, 'e': 1}
Exercise 105 || Solution
Write a function in Python that takes a list of integers as a parameter and returns a dictionary whose keys are the list integers
and whose values are "even" or "odd" depending on the number parity.
Exercise 106 || Solution
Write a program in Python that asks the user to enter ten integers of their choice and return them a dictionary whose keys are the
entered integers and whose values are 'prime' or 'not prime' depending on the entered integer.
Exercise 107 || Solution
Write a program in Python that asks the user to enter ten integers of their choice and return them a dictionary whose keys are the integers entered and whose values are the lists of divisors of the numbers entered. Example if the user enters the numbers: 2, 7, 11, 5, 3, 19, 14, 9, 1, 4, the program returns the dictionary:
d = {2: [1,2], 7: [1,7], 14: [1,2,7,14],
9: [1,3,9], 11: [1,11], 5: [1,5],
3: [1,3], 19: [1,19], 1: [1], 4: [1,2,4]}
Exercise 108 || Solution
Write a python program that asks the user to enter an integer n and return a dictionary whose keys are integers 1, 2, 3, ... n and whose values are 1! , 2! , 3! , … , n!
Exercise 109 || Solution
Write a program in Python that asks the user to enter an integer n and return a dictionary whose keys are the integers 1, 2, 3, ... n and whose values are the sums 1, 1+ 2, 1 + 2 + 3,…, 1 + 2 + 3 +… + n.
Exercise 110 || Solution
Write a Python program that asks the user to enter a text and return a dictionary whose keys are the words of the text entered and the values are the lengths of the words that make up the text. Example for the text T = "Python is a programming language", the program must return the dictionary:
d = {'Python': 6, 'is': 3, 'a': 3, 'language': 7, 'de': 2, 'programming': 13}
Exercise 111 || Solution
Write a Python program that asks the user to enter a text and return him a dictionary whose keys are the words of the text entered and the values are the reverse of the words that make up the text. Example for the text T = "Python is easy", the program must return the dictionary:
d = {'Python': 'nohtyp', 'is': 'si', 'easy': 'ysae'}
Exercise 112 || Solution
Given a dictionary d whose key values are lists. Write a Python program that transforms the dictionary d by sorting the lists. Example for the dictionary:
d = {'a1': [21, 17, 22, 3], 'a2': [11, 15, 8, 13], 'a3': [7, 13, 2, 11], 'a4': [22,14,7,9]}
The program should return the dictionary:
d = {'a1': [3, 17, 21, 22], 'a2': [8, 11, 13, 15], 'a3': [2, 7, 11, 13], 'a4': [7, 9, 14, 22]}
Exercise 113 || Solution
Write a Python program that allows from a given directory to create a dictionary whose keys are the names of the text files located in this directory and the keys are the numbers of the lines of the files.
Exercise 114 || Solution
We consider the Pythons dictionary which contains a personal data :
personalData = {'Name' : 'David' , 'Email' : 'david@gmail.com' , 'Phone' : 33354587 , age' : 27 }
Write a Python program that save data contained in personalData into a file called data.txt .
Exercise 115 || Solution
1) - Write a python program which creates a file named data.txt and write the following lines in this file:
Name: David
Email:
david@gmail.com
Phone: 33354587
age: 27
2) - Write a Python program that reads the data.txt file and exports its content to a dictionary:
d = {'Name': 'David', 'Email': 'david@gmail.com', 'Phone': 33354587, age ': 27}
Exercise 116 || Solution
From the following two lists, create a dictionary by two different methods:
keys = ['Name', 'Email', 'Phone']
values = ['Albert', 'albert@gmail.com', 2225668877]
The output should be:
data = {'Name': 'Albert', 'Email': 'albert@gmail.com', 'Phone': 2225668877}
Exercise 117 || Solution
Write a python algorithm as function that verify if a key is present in given dictionary or note.
Exercise 118 || Solution
The following dictionary contains a student's math scores:
scores = {'score1' : 16, 'score2' : 14, 'score3' : 17}
update the notes dictionary by adding the average of scores.
Exercice 119 || Solution
We cosider the following dictionary of length N containing the students names as keys and their score lists as key values:
d = {'student1': listNote1, 'students2': listNotes2, ..., 'studentN': listNoteN}
Write a Python program that transforms this dictionary by replacing the score lists with their averages.
Example if
d = {'student1': [14, 16, 18], 'students2': [12, 15, 17], 'student3': [16, 16, 13]}
, the program returns:
d = {'student1': 16.0, 'students2': 14.666666666666666, 'student3': 15.0}
Exercise 120 || Solution
Write a python program that transforms a dictionary into an html table and saves the content to an html file called 'convert_dictionary.html'.
Example for the dictionary:
name_email = {'david': 'david@gmail.com', 'hafid': 'hafid@gmail.com', 'nathalie': 'nathalie@gmail.com', 'najib': 'najib @ gmail.com '}
the program should create an html file that displays the content in an html table:
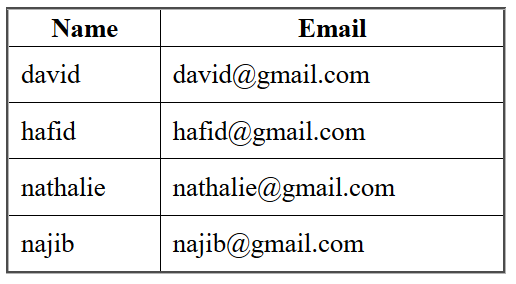
Younes Derfoufi
my-courses.net
------------------------------------------------------------------------ |