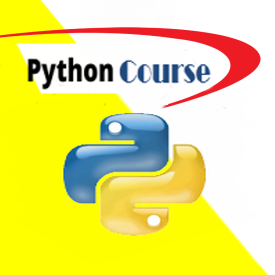
Exercise 89
Write a Python algorithm which determines the list of repeated charcters in given string. Example: if s = "Programming language", the algorithm returns the list:
['r', 'g', 'a', 'm', 'n']
Solution
# create a function to test if given character is repeated within a given string
def isRepeated(s,c):
counter = 0
for x in s:
if x == c:
counter = counter + 1
if counter >= 2:
return True
else:
return False
# create a function that returns the list of all repeated characters
def repeated(s):
# initializing the list of all repeated characters
listRepeated = []
for x in s:
if isRepeated(s , x) and x and x != " " and x not in listRepeated:
listRepeated.append(x)
return listRepeated
# Example
s = "programming language"
print("List of repeated characters in s: " , repeated(s))
# The output is : List of repeated characters in s: ['r', 'g', 'a', 'm', 'n']
Younes Derfoufi
my-courses.net
my-courses.net