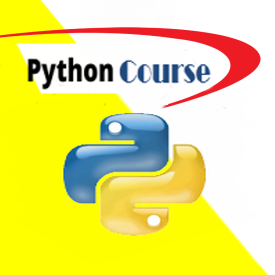
Exercise 35
Write a python algorithm as a function which takes as parameter a string text T and which returns the list of words containing at least one number and one capital letter. Example: if T = 'Python2.7 has been replaced by Python3.X since 2018', the algorithm return: ['Python2.7', 'Python3.X']
Solution
# creating a function which calculates the number of digits on a string
def number_digit (s):
# initialization of the number of digits
nbrDigit = 0
for x in s:
if x.isdigit ():
nbrDigit = nbrDigit + 1
return nbrDigit
# creation of a function which calculates the number of capital letters in a string
def number_maj (s):
# initializing the number of capitals
nbrMaj = 0
for x in s:
if x.isupper ():
nbrMaj = nbrMaj + 1
return nbrMaj
# creating a function which returns the list of words containing at least one number and one uppercase
def listMajDigits (s):
# initialization of the searched words list
listWord = []
# convert string s to a list
L = s.split ()
for word in L:
if number_digit (word)> 0 and number_maj (word)> 0:
listWord.append (word)
return listWord
# Example
T = "Python2.7 has been replaced by Python3.X since 2018"
print (listMajDigits (T)) # the output is: ['Python2.7', 'Python3.X']
Younes Derfoufi
my-courses.net
my-courses.net