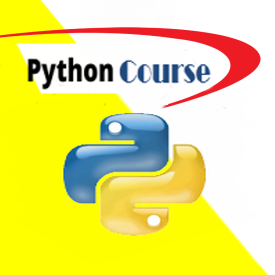
Exercise 49
Write a Python algorithm as a function that takes a list L as a parameter and returns the maximum number of elements with even index without using any predefined functions in Python. Example if L = [13, 2, 31, 120, 4, 97, 15], the algorithm returns the number 31.
Solution
def maxEven (L):
# initialize the maximum of even index elements
evenMax = L [0]
# iterate over even index items in the list
for i in range (0, len (L)):
if i% 2 == 0:
# each time that evenMax < L[i] replace evenMax by L[i]
if evenMax <L [i]:
evenMax = L [i]
return evenMax
# Example
L = [13, 2, 31, 120, 4, 97, 15]
print (maxEven (L)) # output: 31
Younes Derfoufi
my-courses.net
my-courses.net