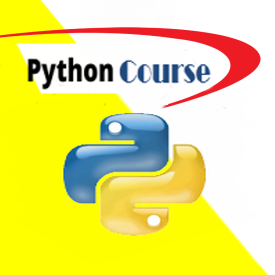
Exercise 77
Write a Python algorithm which determines the first index of an existing occurrence within a given string s without using any predefined method as find() or rfind()... The algorithm must return -1 if the occorrence does not exist within the string s. Example if s = "Python programming language", and occ = "prog" the algorithm return 7
Solution
def findFirstOccurrence(s , occ):
# getting the length of the occurrence occ and the len of the string s
m = len(occ)
n = len(s)
# initialize index
index = -1
# searching the occurrence occ within the string s
for i in range(0 , n-m):
if s[i : m + i] == occ:
index = i
break
return index
# Example:
s = "Python programming language"
occ1 = "prog"
occ2 = "algorithm"
print(findFirstOccurrence(s, occ1)) # display: 7
print(findFirstOccurrence(s, occ2)) # display: -1
Younes Derfoufi
my-courses.net
my-courses.net